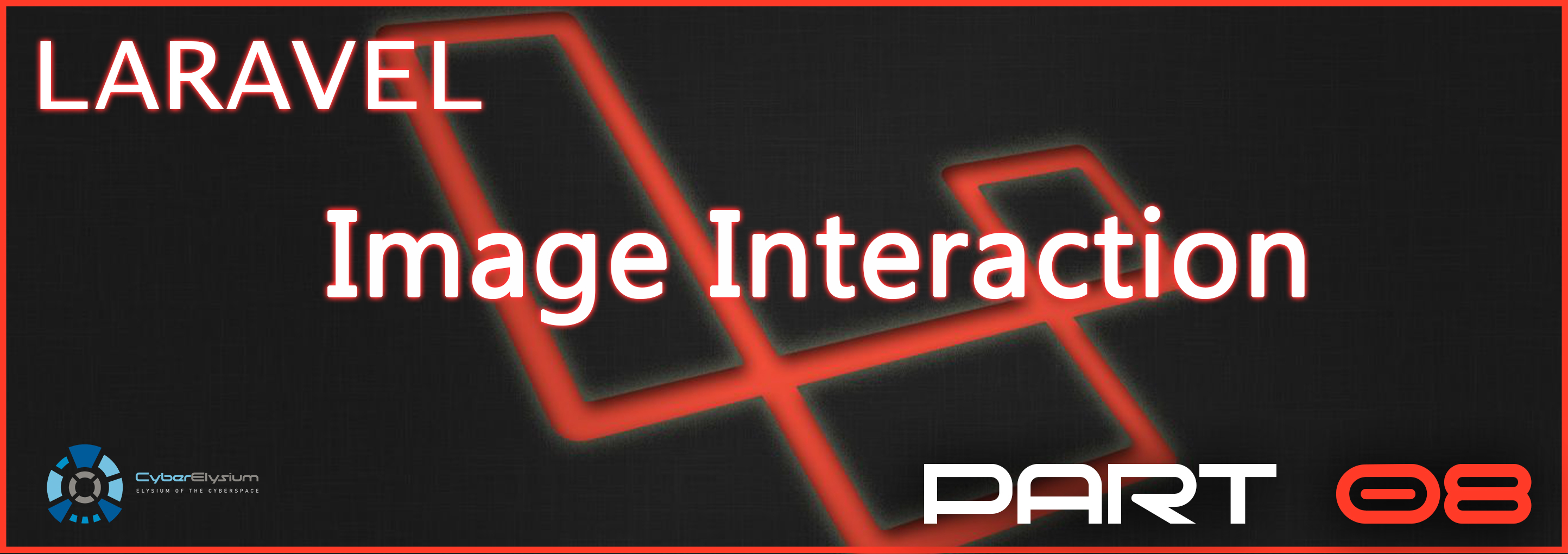
Laravel 8 PHP Tutorial Series
Tutorial 08
Link: PHP Laravel Intermediate tutorial | How to interact with images in Laravel - Tutorial 08 - YouTube
How to interact with images in Laravel
config/images.php
return [
'driver' => env('IMAGE_DRIVER', 'gd'),
'upload_path' => env('IMAGE_UPLOAD_PATH', '/uploads'),
'access_path' => env('IMG_PATH', 'http://todo.cp/uploads'),
1 => ['width' => 35, 'height' => 35],
2 => ['width' => 480, 'height' => 635],
3 => ['width' => 1920, 'height' => 700],
4 => ['width' => 960, 'height' => 960],
5 => ['width' => 105, 'height' => 140],
];
infrastructure/FileService.php
namespace infrastructure;
use Illuminate\Support\Facades\Config;
use Illuminate\Support\Facades\Storage;
class FileService
{
protected $image_path;
public function __construct()
{
$this->image_path = Config::get('images.upload_path');
}
/**
* get file contents of given file
*/
public function getContents($path)
{
return file_get_contents($path);
}
/**
* store given file in the given path
*/
public function storeFile($file, $name, $path, $mode)
{
$fp = fopen(($path . "/" . $name), $mode);
fwrite($fp, $file);
fclose($fp);
}
/**
* Generate new name for uploaded file.
*/
public function makeName($file = null, $url = null)
{
//Returns file name with extension if method contains a file in parameters
if (!($file == null)) {
return md5(date('yyyy-mm-dd hh:ss') . $file . rand(0, 999)) . '.' . $file->getClientOriginalExtension();
}
//Returns file name with extension if method contains a url in parameters
if (!($url == null)) {
return md5(date('yyyy-mm-dd hh:ss') . $file . rand(0, 999)) . '.' . $this->getExt($url);
}
//Returns file name without extension if the $file parameter is null
return md5(date('yyyy-mm-dd hh:ss') . $file . rand(0, 999));
}
/**
* Download the file from url
*/
public function download($url, $name, $target_path)
{
Storage::put($target_path . $name, file_get_contents($url), 'public');
return $name;
}
public function getFilePath()
{
return $this->image_path;
}
public function getExt($file)
{
$ext = pathinfo($file, PATHINFO_EXTENSION);
if (strpos($ext, '?')) {
return substr($ext, 0, strpos($ext, "?"));
} else {
return $ext;
}
}
public function getSize($file)
{
$ch = curl_init($file);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, TRUE);
curl_setopt($ch, CURLOPT_HEADER, TRUE);
curl_setopt($ch, CURLOPT_NOBODY, TRUE);
$size = curl_getinfo($ch, CURLINFO_CONTENT_LENGTH_DOWNLOAD);
curl_close($ch);
return $size;
}
public function getName($file)
{
return pathinfo($file)['basename'];
}
/**
* Validate given extension with file
*/
public function validateExt($file, $ext)
{
if (is_object($file)) {
return $file->extension() == $ext;
} else {
return $this->getExt($file) == $ext;
}
}
}
infrastructure/Images.php
infrastructure/Images.php
namespace Infrastructure;
use App\Models\Image;
use App\User;
use Illuminate\Support\Facades\Config;
use Illuminate\Support\Facades\File;
use infrastructure\Facades\FileFacade;
use Intervention\Image\ImageManager;
class Images
{
protected $image_path;
public function __construct()
{
$this->image_path = public_path(Config::get('images.upload_path'));
}
/**
* Upload the uploaded file to specific path.
*/
public function up($file, $thumb_sizes, $name = null)
{
if ($name) {
$renamed_uploaded_file = FileFacade::makeGivenName($file, $name);
} else {
$renamed_uploaded_file = FileFacade::makeName($file);
}
if ($file->move($this->image_path . '/', $renamed_uploaded_file)) {
foreach ($thumb_sizes as $size) {
$this->saveThumb($renamed_uploaded_file, $size);
}
return $this->makeObject($renamed_uploaded_file);
}
}
public function getThumbSize($size_type)
{
return Config::get('images.' . $size_type);
}
/**
* save image thumbnail
*/
public function saveThumb($file, $size_type)
{
$manager = new ImageManager(array('driver' => Config::get('images.driver')));
$image = $manager->make($this->image_path . '/' . $file);
$size = $this->getThumbSize($size_type);
/**
* resize image with maintaining aspect ratio
*/
if ($image->getWidth() > $image->getHeight()) {
$image->resize(null, $size['height'], function ($image) {
$image->aspectRatio();
});
$image->crop($size['width'], $size['height'], intval(($image->getWidth() - $size['width']) / 2), 0);
} else {
$image->resize($size['width'], null, function ($image) {
$image->aspectRatio();
});
$image->crop($size['width'], $size['height'], 0, intval(($image->getHeight() - $size['height']) / 2));
}
if (!file_exists($this->image_path . '/thumb')) {
File::makeDirectory($this->image_path . '/thumb');
}
if (!file_exists($this->image_path . '/thumb/' . $size['width'] . 'x' . $size['height'])) {
File::makeDirectory($this->image_path . '/thumb/' . $size['width'] . 'x' . $size['height']);
}
$image->save($this->image_path . '/thumb/' . $size['width'] . 'x' . $size['height'] . '/' . $file);
}
/**
* function to delete images from the directory and database
*/
public function delete($id, $sizes)
{
$image = Image::find($id);
File::delete($this->image_path . '/' . $image->name);
foreach ($sizes as $size_type) {
$size = $size = $this->getThumbSize($size_type);
File::delete($this->image_path . '/thumb/' . $size['width'] . 'x' . $size['height'] . '/' . $image->name);
}
Image::destroy($id);
}
/**
* function to delete image from the directory
*/
public function clear($name, $sizes)
{
File::delete($this->image_path . '/' . $name);
foreach ($sizes as $size_type) {
$size = $size = $this->getThumbSize($size_type);
File::delete($this->image_path . '/thumb/' . $size['width'] . 'x' . $size['height'] . '/' . $name);
}
}
/**
* Get image name from uploaded image list string.
*/
public function get($array_list)
{
return explode(',', $array_list);
}
/**
* Download Facebook profile image from profile ID
*/
public function downloadImage($contents, $thumb_sizes)
{
$new_image_name = FileFacade::makeName() . ".jpg";
//Store in the filesystem
FileFacade::storeFile($contents, $new_image_name, $this->image_path, "w");
foreach ($thumb_sizes as $size) {
$this->saveThumb($new_image_name, $size);
}
return $this->makeObject($new_image_name);
}
/**
* Store many of images provided as an array
* Returns stored image names
*/
public function store($images, $thumb_sizes)
{
$response = [];
$status = false;
$created_images = [];
$error = null;
if ($images != null) {
if (is_array($images)) {
foreach ($images as $image) {
$file = $this->up($image, $thumb_sizes);
array_push($created_images, $file);
}
} else {
$created_images = $this->up($images, $thumb_sizes);
}
$status = true;
} else {
$error = "Please add at least one image to upload";
}
$response['status'] = $status;
$response['error'] = $error;
$response['created_images'] = $created_images;
return $response;
}
/**
* Crop image
*/
public function crop($id, array $image_details, array $image_sizes)
{
$file_name = Image::find($id)->name;
$manager = new ImageManager(array('driver' => Config::get('images.driver')));
$image = $manager->make($this->image_path . '/' . $file_name);
$image->crop(
intval($image_details['width']),
intval($image_details['height']),
intval($image_details['x']),
intval($image_details['y'])
);
$image->save($this->image_path . '/' . $file_name);
foreach ($image_sizes as $size) {
$this->saveThumb($file_name, $size);
}
}
/**
* Create Image object and store in database
*/
public function makeObject($new_image_name)
{
$image_service = new Image();
$image = $image_service::create(['name' => $new_image_name]);
return $image;
}
}
domain/Services/BannerService.php -> BannerCreate Function
domain/Services/BannerService.php -> BannerCreate Function
public function store($data)
{
if(isset($data['images'])){
$image = ImagesFacade::store($data['images'], [1,2,3,4,5]);
$data['image_id'] = $image['created_images']->id;
}
$this->banner->create($data);
}
resources/views/pages/banner/index.blade.php -> Banner Upload Form
resources/views/pages/banner/index.blade.php -> Banner Upload Form
<form role="form" action="{{ route('banner.store') }}" method="post" enctype="multipart/form-data">
@csrf
<div class="row justify-content-center">
<div class="col-lg-8">
<div class="form-group">
<input class="form-control" name="title" type="text" placeholder="Enter Banner Title" required>
</div>
<div class="mt-5 form-group">
<input class="form-control dropify" name="images" type="file"
accept="image/jpg, image/jpeg, image/png" required>
</div>
</div>
<div class="mt-4 text-center col-lg-8">
<button type="submit" class="btn btn-success">Submit</button>
</div>
</div>
</form>
CDN Website : https://cdnjs.com/
Facebook : https://www.facebook.com/CyberElysium
Instagram : https://www.instagram.com/cyber_elysium/
Twitter : https://twitter.com/CyberElysium
LinkedIn : https://www.linkedin.com/company/CyberElysium
Facebook Community : https://www.facebook.com/groups/2945714992424838/
Visit our website for more details: https://cyberelysium.com/
Share this post with your friends